The current issue of 2600: The Hacker Quarterly has an article on cryptography that outlines the use of the Vernam Cipher (aka One-time pad) to encrypt text by hand. What makes this technique interesting is its ease of implementation, but even more interesting than that is the fact that the Vernam Cipher is the only known method of encryption that is proven to be unbreakable when implemented/used correctly.
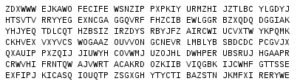
So how does it work? In simple terms, each character from your text, known as plaintext, is encrypted by modular arithmetic with a character from a secret random key (or pad) of the same length as your plaintext. What results is a ciphertext. If the key is TRULY random and as large as the original text and has never been used then the ciphertext will be impossible to decrypt without knowing the key.
This got me to thinking — what would it take to create a web application to easily implement this encryption method for use on data that I wanted to store, let’s say, in my file based wiki!? Sure, there are numerous tools out there that one could leverage to encrypt text but this seemed like an interesting challenge and an excuse to learn a bit more about this cryptography method.
Before I proceed, let me just state that I am not a cryptographer and that the technique I devised for implementing my cipher is for informational purposes only!
The first step to encrypting our text is to convert our letters, and if we choose, our punctuation, to numbers so we can perform our modular arithmetic operations. The 2600 article described what is known as a straddling checkerboard which gives us numeric values for our non-number text characters while simultaneously introducing fractionation.

Using this particular implementation of the straddling checkerboard, Geek Tips would translate into 245527 18607. When encrypting by hand, it’s common to break the data up into smaller, easier to manage, groups. In this case we’ll use groups of five digits. Since our third group is less than five digits, we’ll fill in with zeros. Following this pattern, our string becomes, 24552 71860 70000, which is now ready for the next stage of the ciphering process which is to perform modular arithmetic with a key.
As I stated before, to implement this cipher properly, the key should be completely random and at least as long as our new string of numbers. For this example, let’s say our key is 20247 88641 30412. Now I subtract each digit in the key from each digit in the plaintext. If the result is less than zero, I add 10. For example, in the fifth column, I calculate 2 – 7, which results in -5. Because this is less than zero, I add 10 to get 5 as the final answer. In the first column, the result of 2 – 2 is 0, which is not less than zero, so I leave that as my final answer.
24552 71860 70000 (plaintext) - 20247 88641 30412 (key) ------------------------- 04315 93229 40698 (encrypted)
Decrypting our string is as simple as reversing the modular arithmetic by adding the key to the encrypted string. This will reproduce our original plaintext string. To convert the string back to the original message we simply refer back to our straddling checkerboard grid to recompose the message – SIMPLE!!
The first thing I needed to do to move this project forward was write a simple form to gather my data:
<html> <body> <form action="process.php" method="post"> <p>Your message:<br /><textarea name="text" rows="40" cols="180" /></textarea></p> <p>Decode: <input type="checkbox" name="decode" value="yes" /></p> <p>Enter Key: <input type="password" value="" name="key" autocomplete="off" /></p> <p><input type="submit" value="Encode/Decode" />&nbsp;&nbsp;&nbsp;<input type="reset" value="Reset" /></p> </form> </body> </html>
With my form in place, now I could start developing the code to replicate the straddling checkerboard cipher that was described in the 2600 article. The resulting script was fully functional but WAY more complex than I wanted. As I thought about ways to simplify my code, I recalled that most programming languages have a number of built-in bitwise operators that I might be able to leverage to really simplify what I was trying to accomplish.
The operator that I narrowed in on is called XOR. It works by looking at two bit patterns of equal length and performs a logical exclusive OR operation on each pair of corresponding bits. The result in each position is 1 if only the first bit is 1 OR only the second bit is 1, but will be 0 if both are 0 or both are 1. This is equivalent to being 1 if the two bits are different, and 0 if they are the same.

As this example shows, the 4, 2 and 1 columns have bits in the ON position. Since the 2 column bit is ON for both our numbers it becomes OFF, that leaves the 4 and 1 columns. When we add those together the resulting number is five. Since this XOR functionality is built-in to PHP the complexity of building this computational logic was removed thus allowing me to really simplify my code. Following are the results:
<?php $key = $_POST['key']; // Our plaintext/ciphertext if (strtolower($_POST['decode']) == "yes") { $text = base64_decode($_POST['text']); } else { $text = $_POST['text']; } // Iterate through each character for($i=0;$i<strlen($text);) { for($j=0;$j<strlen($key);$j++,$i++) { $outText .= $text{$i} ^ $key{$j}; } } if (strtolower($_POST['decode']) == "yes") { echo "<p>Your message:<br /><textarea name=\"text\" rows=\"40\" cols=\"180\">" . $outText . "</textarea></p>"; } else { echo "<p>Your encoded message:<br /><textarea name=\"text\" rows=\"40\" cols=\"180\">" . base64_encode($outText) . "</textarea></p>"; } echo "<br /><br /><a href='https://www.example.com/form.html'>Return</a>"; ?>
If the key provided matches the criteria outlined previously then the output from this script should be a true one-time pad. The reality, however is that it’s highly impractical to meet the one-time pad key criteria to make our ciphertext unbreakable. If you choose to use the same key for all of your enciphering (more practical) then the resulting ciphertext is, obviously, less secure. If your key is shorter than your plaintext, thus requiring the key to repeat then the resulting ciphertext is now encoded in what is known as a stream cipher.
I wouldn’t necessarily use the results of this script for anything of significant value but it was nonetheless and interesting exercise for gaining a deeper understanding of ciphers and how they can be implemented for obfuscation purposes in ones own solutions.
Until next time – GEEK OUT!
~GT~